I have a wordclock project.
Before I get there, I need to learn about transistors (see previous article) to handle an external power source for my leds and a shift register to control lots of leds with an Arduino.
Why am I not using the digital pins?
For 2 reasons :
-the digital pins are there to input/output a logic (0/1), not power
-my arduino only has 14 digital pins (I need about 25 output)
So to sum it up, we will use
-an external power source to power on our leds,
-transistors to isolate the external power source from the arduino,
-a shift register (74HC595) to handle 8 outputs at once with only 3 pins.
See below a wiring to use shift registers on two leds (it would be more impressive/significant with 8 leds with I was too lazy to draw it all).
Notice that we also use our external power source to power on the Arduino thru the vin pin.
About transistors, next evolution is to replace the serie of transistors by a transistor array like a uln2803a.
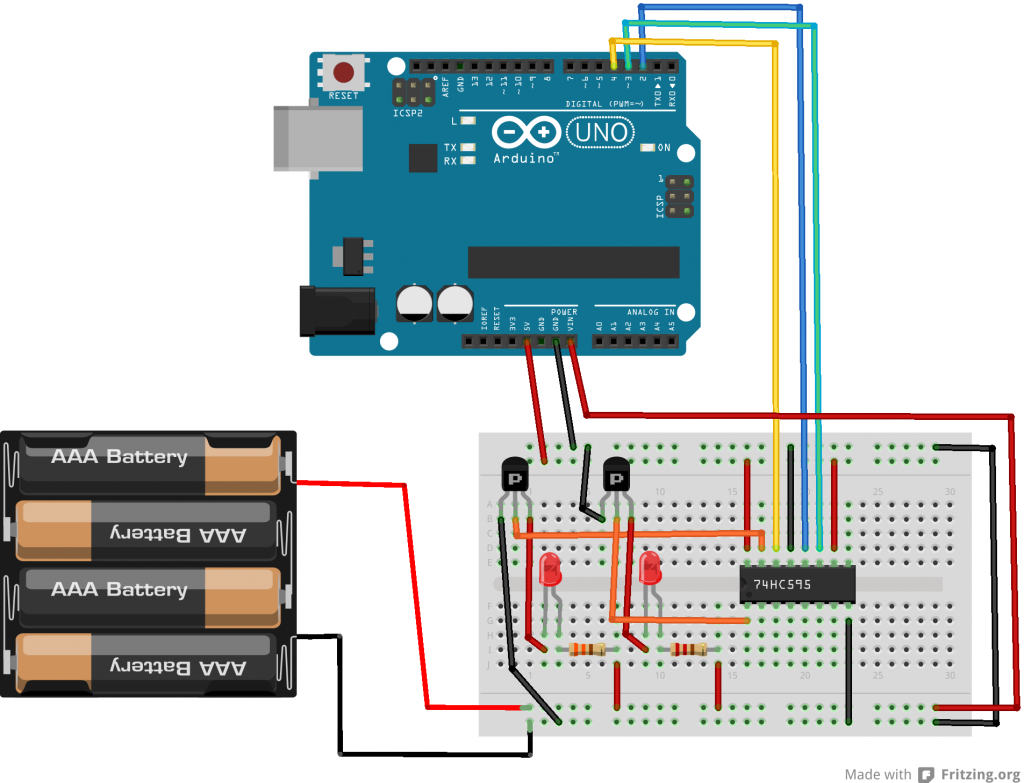
And now the arduino sketch.
note : all led on =b11111111 (255), 7 led on =b0111111, 6 led on=b00111111, etc …
//the pins we are using
int latchPin = 2;
int clockPin = 3;
int dataPin = 4;
void setup() {
//set all the pins used to talk to the chip
//as output pins so we can write to them
pinMode(latchPin, OUTPUT);
pinMode(clockPin, OUTPUT);
pinMode(dataPin, OUTPUT);
}
void loop() {
for (int i = 0; i < 8; i++) {
//take the latchPin low so the LEDs don't change while we are writing data
digitalWrite(latchPin, LOW);
//shift out the bits
shiftOut(dataPin, clockPin, MSBFIRST, i);
//take the latch pin high so the pins reflect
//the data we have sent
digitalWrite(latchPin, HIGH);
// pause before next value:
delay(1000);
}
}